Post details
Command Line toolkit for developing Spring Boot applications
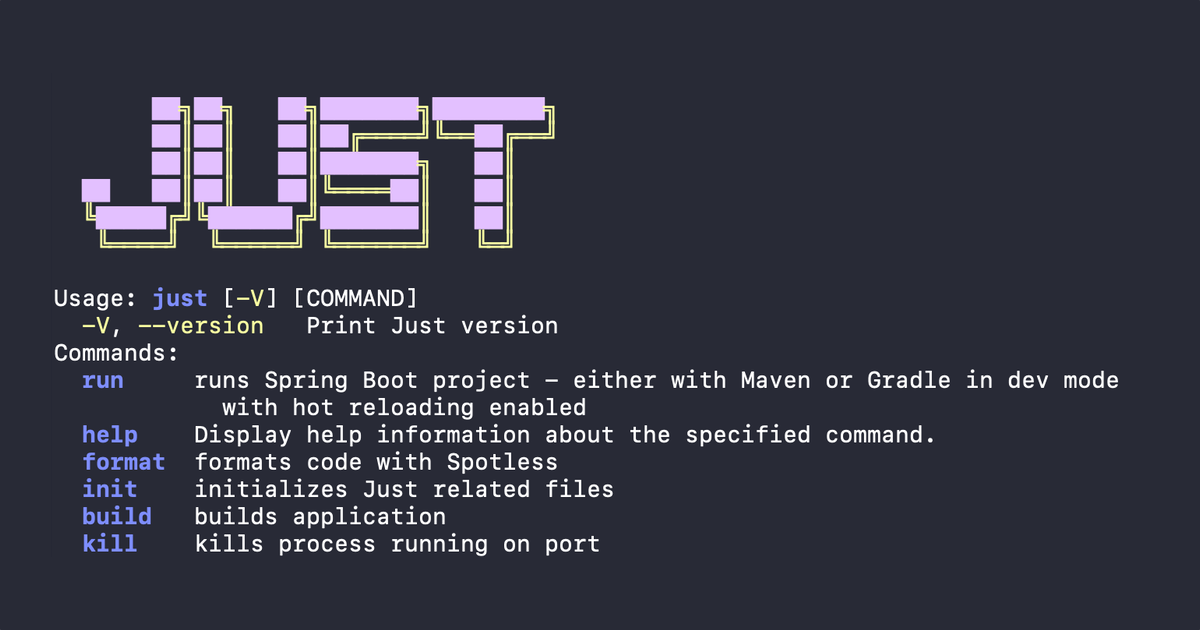
Command Line toolkit for developing Spring Boot applications
<div class="paragraph"> <p>When designing software systems, architects and developers have plenty of architectural options to choose from. Microservice-based systems have become ubiquitous in the last couple of years. However, the idea of monolithic, modular systems has also regained popularity recently. Independent of the architectural style ultimately selected, the individual applications comprising the overall system need their structure to be evolvable and able to follow changes in business requirements.</p> </div> <div class="paragraph"> <p>Traditionally, application frameworks have provided structural guidance by providing abstractions aligned with technical concepts, such as Spring Framework’s stereotype annotations (<code>@Controller</code>, <code>@Service</code>, <code>@Repository</code>, and so on). However, shifting the focus to <a href="https://dannorth.net/2022/02/10/cupid-for-joyful-coding/#domain-based">align code structure with the domain</a> has proven to lead to better structured applications that are ultimately more understandable and maintainable. Until now, the Spring team has given verbal and written guidance on how we recommend structuring your Spring Boot applications. We decided that we can do more than that.</p> </div>
Lessons learned from modernising a lesser maintained (Spring Boot) service (16 mins read).
What I learned from taking ownership of a lesser maintained service and bringing it up to a better standard.
Avoiding Spring context issues when parallelising @Nested
Spring integration tests (3 mins read).
Using abstract base classes to reduce risk of Spring context overall issues with Spring (Boot) integration tests.
Integration Testing Your Spring WebClient
s with Wiremock (4 mins read).
How to write integration tests using Wiremock, for use with WebClient
s.
How to fix Failed to load ApplicationContext
in Spring (Boot) applications (6 mins read).
Some common issues with Spring dependency injection and how to fix them.
Gotcha: checked and unchecked exception handling from Spring WebFlux WebClient
(2 mins read).
How checked and unchecked exceptions may appear when testing your WebClient
code.
Accessing the response body with Spring WebFlux WebClient
with onStatus
(1 mins read).
How to access the body of an (error) response when using WebClient
's onStatus
method.
Converting Spring Boot Property Variables to Environment Variables with Go (1 mins read).
A command-line script to convert a Spring Boot property to an environment variable.
Testing @Scheduled
annotations with Spring (Boot) (2 mins read).
How to test your Spring (Boot) scheduling without waiting for hours.
How to interpolate a property inside Spring Security @PreAuthorize
/ @PostAuthorize
(3 mins read).
How to use the value of a property in a Spring Security authorization statement.
Adding a Wiretap to a Spring WebFlux WebClient
to Log All Request/Response Data (2 mins read).
How to log all request/response data from a Spring Webflux WebClient
.
Determining if the Spring Boot Application is Running in Debug or Trace Mode (3 mins read).
How to determine if your Spring Boot application is running in debug mode, for instance to selectively enable other features of your application.
Simplifying Spring (Boot) ExceptionHandler
s by extending ResponseStatusException
(1 mins read).
How to drive HTTP response status codes from exceptions by extending Spring's ResponseStatusException
.
Excluding Filter
s When using WebMvcTest
(1 mins read).
How to exclude specific Filter
s from running when performing WebMvcTest
s.
Integration Testing Your Spring WebClient
s with okhttp's MockWebServer
(5 mins read).
How to write integration tests using MockWebServer
with Spring Boot, for use with WebClient
s.
Validating a MockMvc
Response Has No Content Type (1 mins read).
How to validate, in a MockMvc
test, whether the response has no content-type
nor a body.
Integration Testing Your Spring RestTemplate
s with RestClientTest
, using spring-test (8 mins read).
How to write integration tests using RestClientTest
with Spring Boot, for use with RestTemplate
and RestTemplateBuilder
s.
Announcing spring-content-negotiator
, a Java Library for Content Negotiation with Spring (1 mins read).
Releasing a new library that can support content negotiation in Spring (Boot) applications, i.e. in Filter
s or ExceptionHandlers
.
Getting Started with jMolecules and the (Classical) Onion Architecture, with a Spring Boot project (6 mins read).
A guided example of converting a Spring Boot project to the Onion Architecture pattern.
Simplifying Spring (Boot) ExceptionHandler
s with ResponseStatus
Annotations (2 mins read).
How to use annotations to drive HTTP response codes from a Spring ExceptionHandler
.
Content Negotiation with Servlet Filter
in Spring (Boot) (3 mins read).
How to perform content-negotiation in a Servlet Filter
, to serve the correct representation of error to a consumer, based on the Accept
header.
Content Negotiation with ControllerAdvice
and ExceptionHandler
s in Spring (Boot) (5 mins read).
How to perform content-negotiation in a Spring ExceptionHandler
, to serve the correct representation of error to a consumer, based on the Accept
header.
Error Handling in (Spring) Servlet Filters (2 mins read).
How to return HTTP errors when a Java Servlet fails with Spring (Boot).
Auditing with Spring Boot Actuator (9 mins read).
How to use Spring Boot Actuator for your audit and business event logging needs.
Adding both an ObjectMapper
and a YAMLMapper
to Spring Boot (2 mins read).
How to have an ObjectMapper
and a YAMLMapper
coexisting in a Spring Boot project's bean dependencies.
Autowiring your controllers automagically when using MockMVC and Spring Cloud Contract (2 mins read).
How to automagically set up your Spring controllers when using MockMVC with Spring Cloud Contract.
Validating a Spring (Boot) Response Matches JSON Schema with MockMVC (3 mins read).
How to perform JSON Schema validation for a Spring (Boot) service's response when testing using MockMVC.
Shift Your Testing Left with Spring Boot Controllers (5 mins read).
How to break down your tests for Spring Boot controllers, which could be used when performing Test Driven Development.
Things I Learned Migrating My Personal APIs To Kubernetes (10 mins read).
What I learned while migrating from a number of Java applications on Virtual Private Servers (VPS) to a Kubernetes cluster.
Testing that your Spring Boot Application Context is Correctly Configured (2 mins read).
How to improve the tests you've got to validate that Spring Boot's context is set up correctly.
Testing Data Serialisation/Deserialization using JsonTest
with Spring Boot (3 mins read).
How to use the @JsonTest
test type in Spring Boot to validate your JSON types correctly serialise/deserialise.
Migrating Your Spring Boot Application to use Structured Logging (6 mins read).
How to make your Spring Boot services more supportable by migrating to JSON-emitting structured logging.
Globally Logging all Spring (Boot) Exceptions (3 mins read).
How to log whenever an exception triggers on an exception handler with Spring.
Spring Boot: 'junit-vintage' failed to discover tests
When Using Only JUnit5 Tests (2 mins read).
How to avoid the error 'junit-vintage' failed to discover tests
when using Spring Boot.
Disabling the logging of Spring Security's Default Security Password (2 mins read).
How to disable Spring Boot logging the generated security password
.
Disabling @Valid
Annotation in a Spring Integration Test (1 mins read).
How to disable @Valid
validation in Spring Integration Tests.
Tomcat May Log Cookies Out-of-the-Box (3 mins read).
Warning you about cookies being logged out-of-the-box, and how to resolve it.
You're currently viewing page 1 of 1, of 48 posts.