How to Use Firebase Firestore as a Realtime Database in Android?
Last Updated :
03 Jun, 2024
Firebase Firestore is the backend database that is used to add, read, update and delete data from Android. But in Firebase Firestore there is a separate method that is used to read data from Firebase Firestore in Realtime Database. In this article, we will read data from Firebase Firestore in Realtime Database. Note that we are going to implement this project using the Java language.Â
What we are going to build in this article?Â
We will be building a simple application in which we will be showing a simple TextView. Inside that TextView, we will be updating the data from Firebase Firestore on a real-time basis.Â
Step by Step Implementation
Step 1: Create a New Project
To create a new project in Android Studio please refer to How to Create/Start a New Project in Android Studio. Note that select Java as the programming language.
Step 2: Connect your app to Firebase
After creating a new project. Navigate to the Tools option on the top bar. Inside that click on Firebase. After clicking on Firebase, you can get to see the right column mentioned below in the screenshot.
Inside that column Navigate to Firebase Cloud Firestore. Click on that option and you will get to see two options on Connect app to Firebase and Add Cloud Firestore to your app. Click on Connect now option and your app will be connected to Firebase. After that click on the second option and now your App is connected to Firebase. After connecting your app to Firebase you will get to see the below screen. Â
After that verify that dependency for the Firebase Firestore database has been added to our Gradle file. Navigate to the app > Gradle Scripts inside that file. Check whether the below dependency is added or not. If the below dependency is not present in your build.gradle file. Add the below dependency in the dependencies section. Â
implementation ‘com.google.firebase:firebase-firestore:22.0.1’
After adding this dependency sync your project and now we are ready for creating our app. If you want to know more about connecting your app to Firebase. Refer to this article to get in detail about How to add Firebase to Android App. Â
Step 3: Working with the AndroidManifest.xml file
For adding data to Firebase we should have to give permissions for accessing the internet. For adding these permissions navigate to the app > AndroidManifest.xml. Inside that file add the below permissions to it. Â
XML
<!--Permissions for internet-->
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
Step 4: Working with the activity_main.xml file
Go to the activity_main.xml file and refer to the following code. Below is the code for the activity_main.xml file.
XML
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/idTVHead"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_margin="5dp"
android:layout_marginTop="30dp"
android:text="Firebase Firestore as Realtime Database"
android:textAlignment="center"
android:textAllCaps="false"
android:textColor="@color/purple_500"
android:textSize="20sp" />
<TextView
android:id="@+id/idTVUpdate"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/idTVHead"
android:layout_marginTop="50dp"
android:text="Updated Text"
android:textAlignment="center"
android:textColor="@color/purple_500"
android:textSize="30sp" />
</RelativeLayout>
Step 5: Working with the MainActivity.java file
Go to the MainActivity.java file and refer to the following code. Below is the code for the MainActivity.java file. Comments are added inside the code to understand the code in more detail.
Java
import android.os.Bundle;
import android.widget.TextView;
import android.widget.Toast;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import com.google.firebase.firestore.DocumentReference;
import com.google.firebase.firestore.DocumentSnapshot;
import com.google.firebase.firestore.EventListener;
import com.google.firebase.firestore.FirebaseFirestore;
import com.google.firebase.firestore.FirebaseFirestoreException;
public class MainActivity extends AppCompatActivity {
// creating a variable for text view.
TextView updatedTV;
// initializing the variable for firebase firestore
FirebaseFirestore db = FirebaseFirestore.getInstance();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// initializing our text view.
updatedTV = findViewById(R.id.idTVUpdate);
// creating a variable for document reference.
DocumentReference documentReference = db.collection("MyData").document("Data");
// adding snapshot listener to our document reference.
documentReference.addSnapshotListener(new EventListener<DocumentSnapshot>() {
@Override
public void onEvent(@Nullable DocumentSnapshot value, @Nullable FirebaseFirestoreException error) {
// inside the on event method.
if (error != null) {
// this method is called when error is not null
// and we get any error
// in this case we are displaying an error message.
Toast.makeText(MainActivity.this, "Error found is " + error, Toast.LENGTH_SHORT).show();
return;
}
if (value != null && value.exists()) {
// if the value from firestore is not null then we are getting
// our data and setting that data to our text view.
updatedTV.setText(value.getData().get("updateValue").toString());
}
}
});
}
}
Step 6: Adding data to the Firebase Firestore console
Go to the browser and open Firebase in your browser. After opening Firebase you will get to see the below page and on this page Click on Go to Console option in the top right corner. Â Â
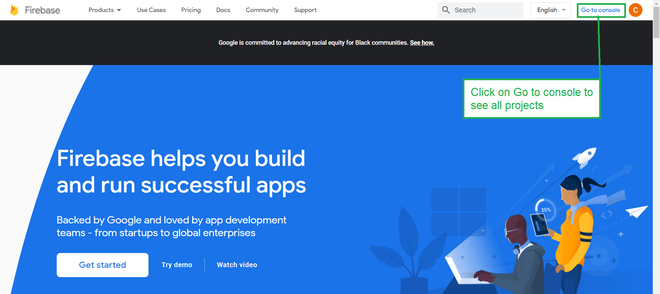
After clicking on this screen you will get to see the below screen with your all project inside that select your project. Â
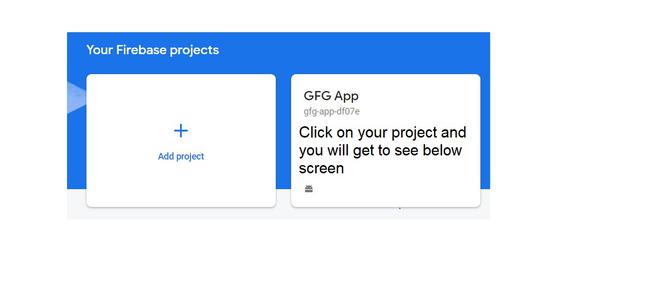
Inside that screen click n Firebase Firestore Database in the left window. Â

After clicking on the Create Database option you will get to see the below screen. Â
Inside this screen, we have to select the Start in test mode option. We are using test mode because we are not setting authentication inside our app. So we are selecting Start in test mode. After selecting on test mode click on the Next option and you will get to see the below screen. Â
Inside this screen, we just have to click on the Enable button to enable our Firebase Firestore database. After completing this process we just have to run our application and add data inside our app and click on the submit button. To add data just click on the Start Collection button and provide the Collection ID as MyData. After that provide the Document ID as Data and inside the Field write down updateValue, and inside the Value provide your Text you want to display. And click on the Save button.
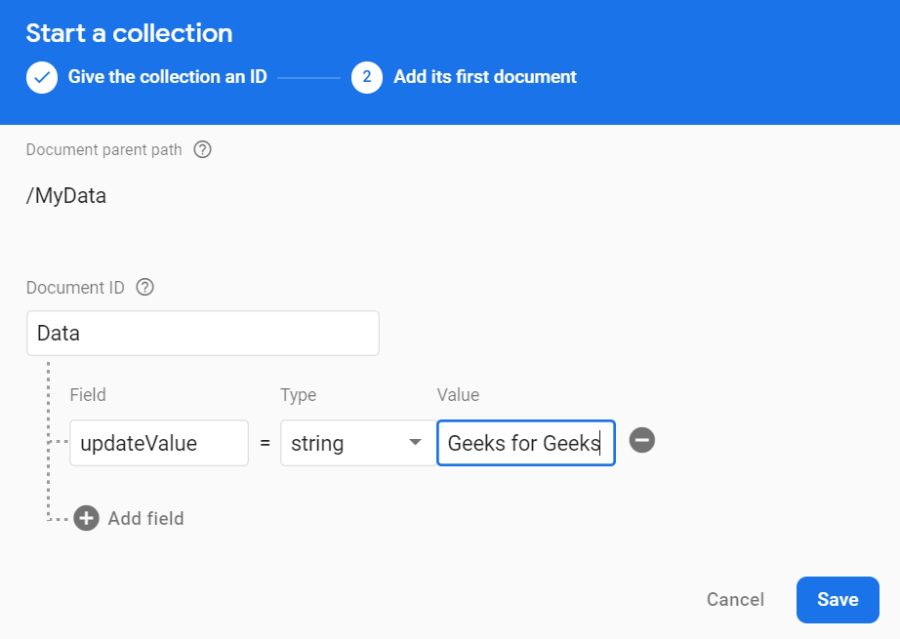
You will get to see the data added inside the Firebase Console. Â
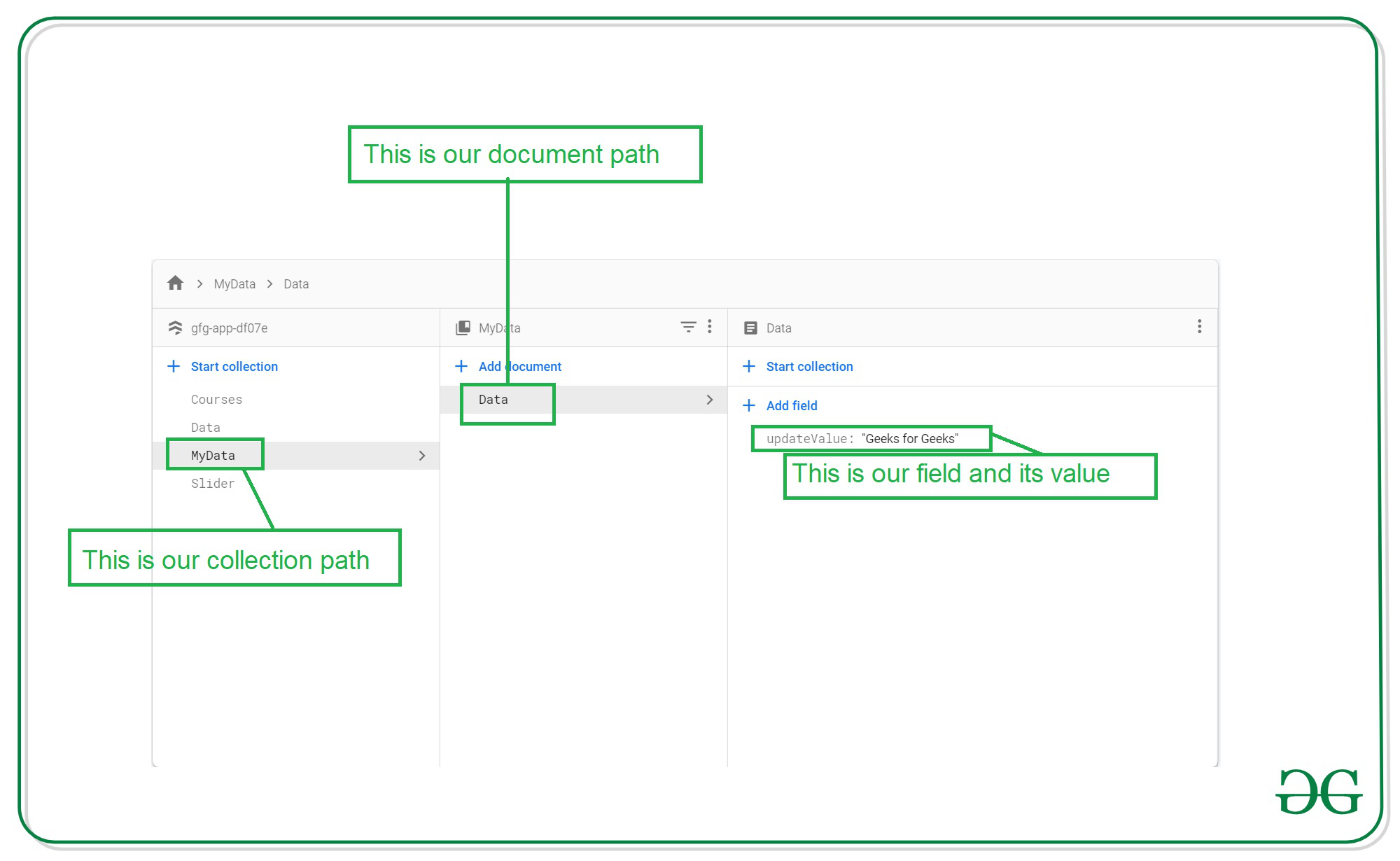
After adding the data to Firebase. Now run your app and see the output of the app. You can change the value in the updateValue field and you can get to see Realtime update on your device.Â
Output:
In the below video we are updating the data when the app is running and it is showing the Realtime Updates in our app.Â